I’ve searched all over the Internet for a Cross Browser JavaScript Copy and Paste solution. I couldn’t find anything that really worked. But I was able to put together the bits and pieces I found into a rather simple solutions. As you may have noticed, I’ve written quite a few articles about programming in JavaScript in the past couple of years because I’ve spent most of my time programming in JavaScript. For the last three months, I’ve been working on a browser based application that needed to be able to copy and paste between the browser and an external spreadsheet. The main struggle in making this all work correct is that what needs to be copied to the clipboard is the underlying data of the application.
Getting this all working in IE, even the most recent version that runs under Windows 10, was pretty easy. And fortunately, the browser of choice at this company is Internet Explorer. But, it is IE 11, which takes twice as long to do anything with JavaScript as Chrome does. Chrome is their secondary browser and my mission has been to find a way to get copy and paste working reliably using Chrome so that the end user would have a better experience with the application.
While the work I’ve been doing has been using Angular, the solution that I provide in this article using plain JavaScript with no dependency on any framework. I want the solution to be available regardless of what framework you might be predisposed to use. If you use angular, or jQuery, or whatever, the code should be easy enough to adapt.
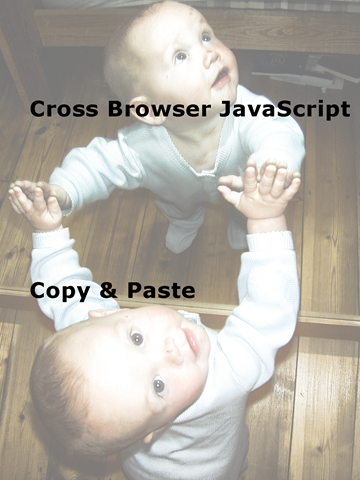
Read More